If you are a server-side Java developer such as myself, I am sure you want an easy way to create AJAX based application but pure JavaScript (ECMAScript) is not your strong skills. The beauty of JavaScript is that it runs on any web server, no need for a servlet container or anything of that sort.
So, I have been writing AJAX based UI using the GWT framework for the past year and half. I am still not a web developer but I understand enough CSS to spice up my site. Anyway, I am writing this tutorial because I think that the GWT Visualization team, even tough they did a good job, over complicates the creation of charts in their tutorial. I was trying to create an Annotated Time Line chart so I looked up my previous code I wrote for the GWT Portlet JSR-168, see here. I also decided to run a search (don't be evil, Google is your friend) to try to find different examples and hopefully some nice custom charts. First of all, based on the search result; I thought that some of examples, including Google's own, were confusing and over complex. Most examples uses AJAXLoader to load the Visualization API, but you shouldn't have to use this. This is the simplest way to create a GWT Annotated Time Line Chart.
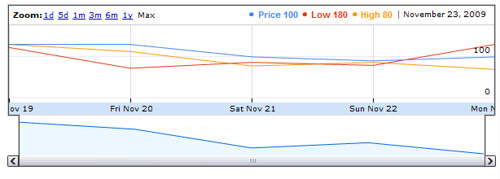
1. Using your favorite development tool with support for GWT framework, create a new Java web application, you can export the compiled JavaScript later to a non-based web application, if you use an IDEW you should code-completion support.
2. Make you sure you have included the GWT Visualization API module in your classpath.
3. In your project source code root directory, you should <AppNamexxxx>.gwt.xml file. make sure to add teh following line to it in order to make the module available to your application.
<inherits name="com.google.gwt.visualization.Visualization"/>
4. In order to keep this tutorial short and straight to point, I have included the chart creation code in my <AppNamexxxx>EntryPoint.java class
Don't forget to import all the necessary classes and voila!
I hope this will save you some time in creating your charts.



So, I have been writing AJAX based UI using the GWT framework for the past year and half. I am still not a web developer but I understand enough CSS to spice up my site. Anyway, I am writing this tutorial because I think that the GWT Visualization team, even tough they did a good job, over complicates the creation of charts in their tutorial. I was trying to create an Annotated Time Line chart so I looked up my previous code I wrote for the GWT Portlet JSR-168, see here. I also decided to run a search (don't be evil, Google is your friend) to try to find different examples and hopefully some nice custom charts. First of all, based on the search result; I thought that some of examples, including Google's own, were confusing and over complex. Most examples uses AJAXLoader to load the Visualization API, but you shouldn't have to use this. This is the simplest way to create a GWT Annotated Time Line Chart.
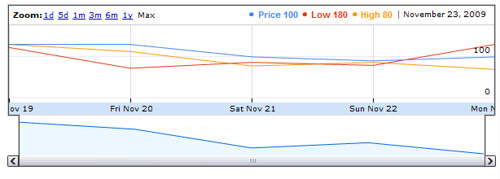
1. Using your favorite development tool with support for GWT framework, create a new Java web application, you can export the compiled JavaScript later to a non-based web application, if you use an IDEW you should code-completion support.
2. Make you sure you have included the GWT Visualization API module in your classpath.
3. In your project source code root directory, you should <AppNamexxxx>.gwt.xml file. make sure to add teh following line to it in order to make the module available to your application.
<inherits name="com.google.gwt.visualization.Visualization"/>
4. In order to keep this tutorial short and straight to point, I have included the chart creation code in my <AppNamexxxx>EntryPoint.java class
public class MainEntryPoint implements EntryPoint
{
/**
* Creates a new instance of MainEntryPoint
*/
public MainEntryPoint()
{
}
/**
* The entry point method, called automatically by loading a module
* that declares an implementing class as an entry-point
*/
public void onModuleLoad()
{
Runnable onLoadCallback = new Runnable()
{
public void run()
{
DataTable data = createHistoryTable(result);
AnnotatedTimeLine.Options options = AnnotatedTimeLine.Options.create();
options.setDisplayAnnotations(true);
options.setDisplayZoomButtons(true);
options.setScaleType(AnnotatedTimeLine.ScaleType.ALLFIXED);
options.setLegendPosition(AnnotatedTimeLine.AnnotatedLegendPosition.SAME_ROW);
AnnotatedTimeLine atl = new AnnotatedTimeLine(data, options, "600px", "200px");
RootPanel.get().add(atl);
}
}
;
VisualizationUtils.loadVisualizationApi(onLoadCallback, AnnotatedTimeLine.PACKAGE);
}
// This method will create the Data used by the chart
private DataTable createHistoryTable()
{
DataTable data = DataTable.create();
data.addColumn(AbstractDataTable.ColumnType.DATE, "Date");
data.addColumn(AbstractDataTable.ColumnType.NUMBER, "Price");
data.addColumn(AbstractDataTable.ColumnType.NUMBER, "Low");
data.addColumn(AbstractDataTable.ColumnType.NUMBER, "High");
data.addRows(5);
DateTimeFormat dtf = DateTimeFormat.getFormat("yyyy-MM-dd");
data.setValue(0, 0, dtf.parse("2009-11-21"));
data.setValue(0, 1, 100);
data.setValue(0, 2, 120);
data.setValue(0, 3, 90);
data.setValue(1, 0, dtf.parse("2009-11-22"));
data.setValue(1, 1, 90);
data.setValue(1, 2, 110);
data.setValue(1, 3, 100);
data.setValue(2, 0, dtf.parse("2009-11-23"));
data.setValue(2, 1, 100);
data.setValue(2, 2, 180);
data.setValue(2, 3, 80);
data.setValue(3, 0, dtf.parse("2009-11-20"));
data.setValue(3, 1, 130);
data.setValue(3, 2, 100);
data.setValue(3, 3, 130);
data.setValue(4, 0, dtf.parse("2009-11-19"));
data.setValue(4, 1, 130);
data.setValue(4, 2, 170);
data.setValue(4, 3, 150);
}
return data;
}
}
Don't forget to import all the necessary classes and voila!
I hope this will save you some time in creating your charts.



The Google API Libraries for Google Web Toolkit is a collection of libraries that provide Java language bindings for popular Google JavaScript APIs. These libraries make it quick and easy for developers to use these Google JavaScript APIs with Google Web Toolkit. The libraries are supported by the Google Web Toolkit team.
ReplyDeletevitamin e
first of all, your code isn't working. theres a superfluid bracket and the dates aren't correct. second, it's absolutely unreadable.
ReplyDeleteThis is a great blog post. Thank you very much for the fantastic insight and we really appreciate the time you took to write this. Thanks again.
ReplyDeleteHouse Buyer San Antonio
Thank you for all of your work on this web page.
ReplyDeleteเว็บบอลออนไลน์
The team is attentive and receptive to feedback, fostering collaboration to produce a final product that exceeds expectations
ReplyDeleteBay Area web site design
I am thankful to you for sharing this plethora of useful information. I found this resource utmost beneficial for me. Thanks a lot for hard work.
ReplyDeleteคาสิโนออนไลน์UFABET
เว็บพนันออนไลน์ufacasino
คาสิโนออนไลน์ที่ดีที่สุด
This is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles
ReplyDeleteเว็บคาสิโนออนไลน์
เว็บพนันคาสิโนออนไลน์
คาสิโนออนไลน์ไม่มีขั้นต่ำ
This is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles
ReplyDeleteeBETเดิมพันขั้นต่ำ10บาท
คาสิโนออนไลน์eBET
เว็บเดิมพันออนไลน์eBET
I conceive you have noted some very interesting points, regards for the post. เล่นบาคาร่าออนไลน์ . บาคาร่าSA Gaming . บาคาร่าSA Gamingดีอย่างไร .
ReplyDeleteThis is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles
ReplyDeleteสมัครสมาชิกAE Casino
ทางเข้าสมัครสมาชิกAE Casino
วิธีเข้าเล่นAE Casino
This is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles
ReplyDeleteสมัครสมาชิกAE Casino
ทางเข้าสมัครสมาชิกAE Casino
วิธีเข้าเล่นAE Casino
Photos available on your site even though producing attention rapidly some quantity submits. eBETเดิมพันขั้นต่ำ10บาท . ทางเข้าคาสิโนออนไลน์eBET . สมัครสมาชิกeBET
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. อีเบท . eBETGaming . รีวิวเว็บพนันeBET
ReplyDeleteI conceive you have noted some very interesting points, regards for the post อีเบท . eBETGaming . รีวิวเว็บพนันeBET
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteI am thankful to you for sharing this plethora of useful information. I found this resource utmost beneficial for me. Thanks a lot for hard work.
ReplyDeletesa sexy gaming
SAsexy
sagamingดีไหม
Wohh just what I was looking for, regards for posting. Venus Casinoดีไหม วีนัสคาสิโนดีอย่างไร คาสิโนวีนัส
ReplyDeleteWohh just what I was looking for, regards for posting. คาสิโนออนไลน์eBET เว็บเดิมพันออนไลน์eBET รีวิวเว็บพนันeBET
ReplyDeleteI conceive you have noted some very interesting points, regards for the post... ทางเข้าVenus Casino . สมัครสมาชิกVenus Casino . วิธีเดิมพันVenus Casino
ReplyDeleteWohh just what I was looking for, regards for posting. เว็บแทงบอลออนไลน์ยูฟ่าเบท
ReplyDeleteWe help them as much as we can. If they need assistance with research, writing, grammar, formatting or proofreading, we provide our assistance to help them build their career. อีเบท. คาสิโนออนไลน์eBET. สมัครeBETCasino
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work.. วิธีเล่นบาคาร่าออนไลน์ . สมัครแทงบาคาร่าออนไลน์ . สมัครสมาชิกเว็บแทงบาคาร่า
ReplyDeleteWohh just what I was looking for, regards for posting. คาสิโนเว็บUFABET
ReplyDeleteI value the article.Thanks Again. Much obliged. วิธีแทงเสือมังกรบนมือถือ ทางเข้าเว็บแทงเสือมังกรออนไลน์ ลิงค์ทางเข้าเล่นเสือมังกรออนไลน์
ReplyDeleteI will bookmark your site and take the feeds additionally. คาสิโนGDG. Gold Diamond Gaming. GDGเดิมพันไม่มีขั้นต่ำ.
ReplyDeleteI value the article.Thanks Again. Much obliged. แทงบาคาร่าขั้นต่ำ10บาท. เว็บพนันบาคาร่าออนไลน์. บาคาร่าออนไลน์บนมือถือ.
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. เสือมังกรออนไลน์
ReplyDeleteThis is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also แทงเสือมังกรออนไลน์.
ReplyDeleteWohh just what I was looking for, regards for posting. Sexy Baccarat
ReplyDeleteWe provide best services to all class of clients. สมัครสมาชิกSexy Baccarat.
ReplyDeleteWohh just what I was looking for, regards for posting. เดิมพันคาสิโนSA .
ReplyDeleteI conceive you have noted some very interesting points, regards for the post.
ReplyDeleteสมัครสมาชิกAE Casino
I conceive you have noted some very interesting points, regards for the post. AE Casinoดีมั้ย?
ReplyDeleteI will bookmark your site and take the feeds additionally เล่นAE Casinoบนมือถือ
ReplyDeleteThis is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles
ReplyDeleteVenus Casino
รีวิวเว็บVenus Casino
This is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles eBETGaming.
ReplyDeleteWe help them as much as we can. If they need assistance with research, writing, grammar, formatting or proofreading, we provide our assistance to help them build their career. รีวิวเว็บพนันeBET.
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. Venus CasinoCasino
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. แนะนำเว็บVenus Casino.
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. เล่นวีนัสคาสิโนบนมือถือ
ReplyDeleteI went to this website, and I believe that you have a plenty of excellent information, I have saved your site to my bookmarks. ทางเข้าGold Diamond Gaming.
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits. เว็บแทงบาคาร่าออนไลน์.
ReplyDeleteI value the article.Thanks Again. Much obliged. บาคาร่าออนไลน์บนมือถือ.
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. เซ็กซี่บาคาร่า .
ReplyDeleteI value the article.Thanks Again. Much obliged. บาคาร่าออนไลน์
ReplyDeleteWohh just what I was looking for, regards for posting. บาคาร่าออนไลน์
ReplyDeleteWohh just what I was looking for, regards for posting. เสือมังกรขั้นต่ำ 10 บาท.
ReplyDeleteI value the article.Thanks Again. Much obliged.
ReplyDeleteเล่นเสือมังกร
I read this article. I think You put a lot of effort to create this article. I appreciate your work. ไพ่เสือมังกร
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits.
ReplyDeleteคาสิโน AE Sexy
This is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also
ReplyDeleteเว็บไซต์เล่นยูฟ่าสล็อตออนไลน์บนมือถือ
UFA SLOT
We provide best services to all class of clients. เกมสล็อตน่าเล่นjoker เกมสล็อตโจ๊กเกอร์ออนไลน์ แนะนำเว็บสล็อตJokerดีที่สุด
ReplyDeleteI value the article.Thanks Again. Much obliged.
ReplyDeleteเล่นยูฟ่าคาสิโน
This is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative. 138BET. สมัครเว็บพนัน138BET. ทางเข้าเว็บพนัน138BET.
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. คาสิโนขั้นต่ำ10บาท
ReplyDeleteI will bookmark your site and take the feeds additionally
ReplyDeleteสมัครสมาชิกSexy Baccarat
I read this article. I think You put a lot of effort to create this article. I appreciate your work. เดิมพันออนไลน์Venus Casino
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. คาสิโนออนไลน์บนมือถือ
ReplyDeleteI went to this website, and I believe that you have a plenty of excellent information, I have saved your site to my bookmarks.
ReplyDeleteSA Gamingออนไลน์
เว็บไซต์SA Gaming
I will bookmark your site and take the feeds additionally.. Sexy gaming .
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. SA GAMING
ReplyDeleteWe help them as much as we can. If they need assistance with research, writing, grammar, formatting or proofreading, we provide our assistance to help them build their career.
ReplyDeleteเว็บไซต์เล่นยูฟ่าสล็อตออนไลน์บนมือถือ
เดิมพันสล็อตUFABET
We help them as much as we can. If they need assistance with research, writing, grammar, formatting or proofreading, we provide our assistance to help them build their career. เล่นเสือมังกรบนมือถือ.
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. เว็บคาสิโนออนไลน์.
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. เล่นคาสิโนeBET
ReplyDeleteWe provide best services to all class of clients. แทงบอลลีกเอิงเว็บไหนดี แทงบอลออนไลน์ที่ดีที่สุด แทงบอลลีกเอิงออนไลน์
ReplyDelete
ReplyDeleteI will bookmark your site and take the feeds additionally คาสิโนขั้นต่ำ10บาท .
I conceive you have noted some very interesting points, regards for the post.คาสิโนขั้นต่ำ10บาท
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. คาสิโนขั้นต่ำ10บาท คาสิโนเซ็กซี่บาคาร่า
ReplyDeleteWohh just what I was looking for, regards for posting.คาสิโนขั้นต่ำ10บาท.วิธีเล่นคาสิโนSexy Baccarat
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. เล่นคาสิโนSA . เดิมพันSA GAME .
ReplyDeleteI value the article.Thanks Again. Much obliged. เล่นsagamingบนมือถือ เดิมพันคาสิโนออนไลน์SA
ReplyDeleteThis is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles. sa sexy gaming. SAsexy.
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work.
ReplyDeleteทางเข้าsagaming .
สมัครสมาชิกsagaming .
I value the article.Thanks Again. Much obliged. วิธีเดิมพันเว็บsagaming เล่นSA GAME
ReplyDeleteI value the article.Thanks Again. Much obliged. คาสิโนขั้นต่ำ10บาท. เว็บคาสิโนออนไลน์
ReplyDeleteI conceive you have noted some very interesting points, regards for the post.
ReplyDeleteวีนัสคาสิโนออนไลน์ฝากถอนAUTO
เว็บVenus Casino
Venus Casinoเดิมพันไม่มีขั้นต่ำ
We provide best services to all class of clients. คาสิโนออนไลน์
ReplyDeleteI will bookmark your site and take the feeds additionally
ReplyDeleteเว็บไซต์เล่นยูฟ่าสล็อตออนไลน์บนมือถือ
เว็บไซต์UFASLOT
เดิมพันสล็อต
I conceive you have noted some very interesting points, regards for the post. เดิมพันAEขั้นต่ำ10บาท.AE Casinoฝาก-ถอนออโต้.AE Casinoเดิมพันไม่มีขั้นต่ำ
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteI am thankful to you for sharing this plethora of useful information. I found this resource utmost beneficial for me. Thanks a lot for hard work.
ReplyDeleteเดิมพันAEขั้นต่ำ10บาท.
AE Casinoฝาก-ถอนออโต้.
AE Casinoเดิมพันไม่มีขั้นต่ำ.
I will bookmark your site and take the feeds additionally. คาสิโนออนไลน์
ReplyDeleteWohh just what I was looking for, regards for posting. ทางเข้ายูฟ่าเบท ทางเข้าเล่นเว็บยูฟ่าเบท
ReplyDeleteI value the article.Thanks Again. Much obliged. เดิมพันยูฟ่าเบทบนมือถือ สมัครufabetเว็บตรง
ReplyDeleteI went to this website, and I believe that you have a plenty of excellent information, I have saved your site to my bookmarks.
ReplyDeleteทางเข้าเว็บพนัน138BET.
138BETTHAILAND.
138BET.
We provide best services to all class of clients. สูตรแทงบอลไหลตามน้ำ สูตรแทงบอล สูตรแทงบอลฝากไม่มีขั้นต่ำ
ReplyDeleteWohh just what I was looking for, regards for posting. ยูฟ่าเบท . เว็บพนันufabet .
ReplyDeleteI value the article.Thanks Again. Much obliged. Ufabet.ยูฟ่าเบท
ReplyDeleteWohh just what I was looking for, regards for posting. Ufabet . ยูฟ่าเบท .
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. ทางเข้าเล่นเว็บยูฟ่าเบท วิธีสมัครสมาชิกufabet
ReplyDeleteI will bookmark your site and take the feeds additionally.ข้อดีของเว็บยูฟ่าเบท.เล่นยูฟ่าเบท
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits. ยูฟ่าเบท . เว็บพนันufabet
ReplyDeleteI unquestionably esteeming each and every piece of it and I have you bookmarked to look at new. ทางเข้าเล่นเว็บยูฟ่าเบท. วิธีสมัครสมาชิกufabet.
ReplyDeleteWe provide best services to all class of clients. วิธีสมัครสมาชิกufabet . วิธีเข้าเล่นufabet
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. เว็บพนันufabet. เดิมพันออนไลน์ufabet.
ReplyDeleteWohh just what I was looking for, regards for posting. แทงบอลเงินสด. เดิมพันบอลเงินสด. เว็บแทงบอลเงินสด.
ReplyDeleteWohh just what I was looking for, regards for posting. กำถั่วขั้นต่ำ10บาท กำถั่วpantip
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits.
ReplyDeleteสูตรสล็อตออนไลน์
รีวิวUFASLOT
เกมสล็อตออนไลน์บนมือถือ
I read this article. I think You put a lot of effort to create this article. I appreciate your work.
ReplyDeleteเทคนิคเล่นUFACASINO
วิธีเล่นคาสิโนUfabet
เดิมพันคาสิโนออนไลน์
I am thankful to you for sharing this plethora of useful information. I found this resource utmost beneficial for me. Thanks a lot for hard work. รีวิวเว็บพนันUFABET
ReplyDeleteWohh just what I was looking for, regards for posting.เล่นเกมกำถั่วออนไลน์.รีวิวเว็บเกมกำถั่ว
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. กำถั่ว . Fantan .
ReplyDeleteI value the article.Thanks Again. Much obliged. กำถั่วขั้นต่ำ10บาท.กำถั่วpantip
ReplyDeleteI value the article.Thanks Again. Much obliged. รีวิวคาสิโนUFABET
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteWohh just what I was looking for, regards for posting. กำถั่วขั้นต่ำ10บาท . กำถั่วpantip .
ReplyDeleteThis is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also กำถั่วขั้นต่ำ10บาท. กำถั่วpantip.
ReplyDeleteWe help them as much as we can. If they need assistance with research, writing, grammar, formatting or proofreading, we provide our assistance to help them build their career. UFABET. เว็บคาสิโนยูฟ่าเบท.
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. สมัครสมาชิคคาสิโนยูฟ่าเบท . เล่นUFA CASINO .
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. ยูฟ่าเบทคาสิโนออนไลน์ คาสิโนยูฟ่าเบทออนไลน์บนมือถือ
ReplyDeleteThis comment has been removed by the author.
ReplyDelete"I read this article. I think You put a lot of effort to create this article. I appreciate your work. ufaslot
ReplyDelete. สล็อตออนไลน์ยูฟ่าเบท . "
I conceive you have noted some very interesting points, regards for the post. แนะนำเว็บufaslot.ยูฟ่าสล็อตฝากไม่มีขั้นต่ำ
ReplyDeleteI went to this website, and I believe that you have a plenty of excellent information, I have saved your site to my bookmarks. UFA SLOT. ยูฟ่าสล็อต.
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. เว็บพนันออนไลน์ufaslot. เล่นufaslotบนมือถือ.
ReplyDeleteWohh just what I was looking for, regards for posting.
ReplyDeleteคาสิโนSA GamingฝากถอนAUTO
เล่นSA Gamingบนมือถือ
เว็บSA Gaming
I am thankful to you for sharing this plethora of useful information. I found this resource utmost beneficial for me. Thanks a lot for hard work. ufaslot . สล็อตออนไลน์ยูฟ่าเบท
ReplyDeleteI unquestionably esteeming each and every piece of it and I have you bookmarked to look at new แทงบอลออนไลน์UFABET
ReplyDeleteWe provide best services to all class of clients. แทงบอลเซเรียอาเว็บไหนดี พนันบอลเซเรียอา แทงบอลเซเรียอายูฟ่าเบท
ReplyDeleteWe provide best services to all class of clients. เดิมพันรูเล็ตขั้นต่ำ10บาท. ทางเข้าเว็บรูเล็ตออนไลน์.
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits. เล่นรูเล็ตออนไลน์ . เล่นรูเล็ต
ReplyDeleteI value the article.Thanks Again. Much obliged. 1XBET.
ReplyDeleteThis is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles. แนะนำเว็บแทงบอล.
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. เกมยิงปลาออนไลน์ . เล่นเกมยิงปลา
ReplyDeleteI conceive you have noted some very interesting points, regards for the post.เดิมพันเกมยิงปลาออนไลน์ เล่นเกมยิงปลาออนไลน์
ReplyDeleteI value the article.Thanks Again. Much obliged. บาคาร่าเว็บไหนดี2021
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. เดิมพันคาสิโนยูฟ่าเบท คาสิโนยูฟ่าเบท2021
ReplyDeleteWohh just what I was looking for, regards for posting.เล่นเกมยิงปลา.เล่นเกมยิงปลาเว็บไหนดี
ReplyDeleteWohh just what I was looking for, regards for posting. รีวิวเว็บพนันบอล.
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. Joker Gaming .
ReplyDeleteWohh just what I was looking for, regards for posting. ข้อดีของเว็บยูฟ่าเบท
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. ทางเข้ายูฟ่าเบท
ReplyDeleteI value the article.Thanks Again. Much obliged.เล่นยูฟ่าเบท
ReplyDeleteI value the article.Thanks Again. Much obliged. https://ufatips.wiki/
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. แทงบอลUFABET
ReplyDeleteI value the article.Thanks Again. Much obliged. ทางเข้าแทงบอลเว็บยูฟ่าเบท
ReplyDeleteWe provide best services to all class of clients. รีวิวคาสิโนUFABET
ReplyDeleteWohh just what I was looking for, regards for posting. สมัครเว็บพนัน138BET.
ReplyDeleteI value the article.Thanks Again. Much obliged.แทงบอลufabetเว็บไหนดี? top10 เว็บแทงบอลที่ดีที่สุด
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits. เกมคาสิโนสด1XBET.
ReplyDeleteI value the article.Thanks Again. Much obliged.
ReplyDeleteSlot Online Joker Gaming UFASLOTออนไลน์
This is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also วิธีเล่นบาคาร่า บาคาร่าเว็บไหนดี2021 วิธีเล่นบาคาร่าufabet
ReplyDelete
ReplyDeleteI will bookmark your site and take the feeds additionally. สมัครเว็บพนันUFABET . สมัครเว็บยูฟ่าเบท .
I value the article.Thanks Again. Much obliged. Sexy gaming
ReplyDeleteI value the article.Thanks Again. Much obliged.
ReplyDeleteทางเข้าคาสิโนออนไลน์
This is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also Sexy Baccarat
ReplyDeleteWe provide best services to all class of clients.
ReplyDeleteคาสิโนออนไลน์ไม่มีขั้นต่ำ.
sexybaccarat365s.com.
Wohh just what I was looking for, regards for posting. คาสิโนออนไลน์บนมือถือ
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. คาสิโนออนไลน์ยูฟ่าเบท
ReplyDeleteI will bookmark your site and take the feeds additionally. UFABET.
ReplyDeleteI will bookmark your site and take the feeds additionally แทงบอลUFABET
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits. UFABET
ReplyDeleteI conceive you have noted some very interesting points, regards for the post.
ReplyDeleteเล่นKingmakerบนมือถือ
This is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also Kingmaker
ReplyDeleteWe provide best services to all class of clients. เล่นคาสิโนคิงเมคเกอร์ออนไลน์
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. แทงบอลได้เงินจริง
ReplyDeleteI conceive you have noted some very interesting points, regards for the post.
ReplyDeleteทางเข้าเดิมพันกีฬายูฟ่าเบท
I value the article.Thanks Again. Much obliged. พนันกีฬาขั้นต่ำ10บาท.
ReplyDeleteWe provide best services to all class of clients. สล็อตออนไลน์
ReplyDeleteI am thankful to you for sharing this plethora of useful information. I found this resource utmost beneficial for me. Thanks a lot for hard work. เล่นสล็อตบนมือถือ
ReplyDeleteI value the article.Thanks Again. Much obliged. สล็อตออนไลน์ฝากไม่มีขั้นต่ำ
ReplyDeleteWe provide best services to all class of clients. พนันกีฬาออนไลน์ยูฟ่าเบท
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work. สมัครสมาชิกSexy Baccarat สมัครสมาชิก
ReplyDeleteI value the article.Thanks Again. Much obliged. สมัครสมาชิกเล่นเซ็กซี่บาคาร่าบนมือถือ
ReplyDeleteI unquestionably esteeming each and every piece of it and I have you bookmarked to look at new stuff you post. สมัครสมาชิกUFABET
ReplyDeleteThis is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also. บาคาร่าออนไลน์
ReplyDeleteThis is very Amazing and Very Informative Artical we get alot of Informations from this artical we really appreciate your team work keep it up and keep posting such a informative articles. ทางเข้าUFABET
ReplyDeleteI value the article.Thanks Again. Much obliged. ทางเข้าเว็บแทงบอลUFABET
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. เล่นบาคาร่าขั้นต่ำ10บาท
ReplyDeleteI will bookmark your site and take the feeds additionally.รีวิวเว็บเล่นบาคาร่า
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. แทงไฮโลขั้นต่ำ10บาท
ReplyDeleteI value the article.Thanks Again. Much obliged. ทางเข้าแทงไฮโลออนไลน์ .
ReplyDeleteI value the article.Thanks Again. Much obliged. เดิมพันไฮโลออนไลน์
ReplyDeleteWe provide best services to all class of clients. PGSLOT GAME
ReplyDeleteI unquestionably esteeming each and every piece of it and I have you bookmarked to look at new เล่นเกมรูเล็ตออนไลน์
ReplyDeleteWohh just what I was looking for, regards for posting. เล่นรูเล็ตออนไลน์ยูฟ่าเบท
ReplyDeleteI value the article.Thanks Again. Much obliged. สมัครเล่นรูเล็ตออนไลน์ .
ReplyDeleteWohh just what I was looking for, regards for posting.
ReplyDeleteRoulette online
I conceive you have noted some very interesting points, regards for the post . Joker Gaming
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work.
ReplyDeleteSpade gaming Slot Online
Photos available on your site even though producing attention rapidly some quantity submits.
ReplyDeleteเว็บแทงหวย
We provide best services to all class of clients. แทงบอลเต็งUFABET
ReplyDeleteI read this article. I think You put a lot of effort to create this article. I appreciate your work.
ReplyDeleteแทงไฮโลเว็บไหนดี
I will bookmark your site and take the feeds additionally.แทงไฮโลเว็บไหนดี
ReplyDeleteWe provide best services to all class of clients.
ReplyDeleteสมัครUFABET
Wohh just what I was looking for, regards for posting. เล่นสล็อตบนมือถือ
ReplyDeleteWe provide best services to all class of clients.
ReplyDeleteแทงบอลไทยufa365ดีมั้ย?
แทงบอลไทยพรีเมียร์ลีก
เดิมพันบอลไทย
I value the article.Thanks Again. Much obliged. เว็บSexy Baccarat คาสิโนสดSexy Baccarat
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. เล่นรูเล็ตออนไลน์เว็บไหนดี
ReplyDeleteWohh just what I was looking for, regards for posting.
ReplyDeleteไพ่เสือมังกร
Wohh just what I was looking for, regards for posting.
ReplyDeleteแทงบอลฝากถอนไม่มีขั้นต่ำ
Photos available on your site even though producing attention rapidly some quantity submits. สมัครเล่นยูฟ่าเบทบนมือถือ
ReplyDeleteI conceive you have noted some very interesting points, regards for the post. สมัครสมาชิกufabet
ReplyDeletePhotos available on your site even though producing attention rapidly some quantity submits.ไฮโลออนไลน์
ReplyDeleteWohh just what I was looking for, regards for posting.แทงบอลออนไลน์ .เว็บพนันบอลUFABET .แทงบอลไทยออนไลน์
ReplyDeleteThis is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also. สล็อตออนไลน์ขั้นต่ำ1บาท
ReplyDeleteThis is a great web site, Good sparkling user interface and, very informative blogs. thanks. You may check our website also.เว็บUFACASINO
ReplyDeleteWe provide best services to all class of clients
ReplyDeleteกติกาเล่นรูเล็ต
I value the article.Thanks Again. Much obliged.
ReplyDeleteคาสิโนออนไลน์